Ⅰ |
This article along with all titles and tags are the original content of AppNee. All rights reserved. To repost or reproduce, you must add an explicit footnote along with the URL to this article! |
Ⅱ |
Any manual or automated whole-website collecting/crawling behaviors are strictly prohibited. |
Ⅲ |
Any resources shared on AppNee are limited to personal study and research only, any form of commercial behaviors are strictly prohibited. Otherwise, you may receive a variety of copyright complaints and have to deal with them by yourself. |
Ⅳ |
Before using (especially downloading) any resources shared by AppNee, please first go to read our F.A.Q. page more or less. Otherwise, please bear all the consequences by yourself. |
|
This work is licensed under a Creative Commons Attribution-NonCommercial-ShareAlike 4.0 International License. |
97 Things Every Programmer Should Know is co-written by 73 different authors working in the software industry. The content is some of their experiences summed up in their work. Based on their practical experience in various aspects of software engineering, they expressed their own opinions and put forward their own insights. These experiences cover many aspects such as user requirements, system analysis and design, coding practices, coding style, bug management and project management. Programmers from all fields can find content of interest to them, so this book is suitable for reading by programmers at different levels.
The great thing about this book is that it doesn’t just apply to Java programming. Of course, some chapters cover Java, but there are other topics as well. Things like understanding your container environment, how to deliver software faster and better, and not hiding your development tools apply to any language development. Even better, some chapters apply equally to real-life issues. Breaking problems and tasks into small pieces is good advice for solving any problem; building a diverse team is important for all collaborators; going from loose pieces to a finished piece looks like a jigsaw player’s train of thought , but also applies to different job roles.
Each chapter is only a few pages long, and there are 97 chapters in total, so you can easily skip chapters that don’t apply to you. Whether you have been writing Java code, have only learned a little Java, or have not yet started learning Java, this will be a good book for geeks interested in code and the software development process. Its greatest value lies in the inspiration it can bring, rather than the specific practices it says. Finally, I strongly recommend that you read this book again every once in a while, so that you will have different gains.
This is a collection post for the title of 97 Things Every Programmer Should Know. AppNee shared the 3 versions of this book here: 97 Things Every Programmer Should Know, 97 Things Every Programmer Should Know – Extended, and 97 Things Every Programmer Should Know (GitBook version). They were written or organized by Kevlin Henney, Shirish Padalkar, and Dmitry Semigradsky respectively.
97 Things Every Programmer Should Know – Extended is the extended version of 97 Things Every Programmer Should Know. 97 Things Every Programmer Should Know contains amazing collection of essays about programming practices, while this book is a collection of additional 68 essays available at the site but doesn’t appear in Kevlin’s book.
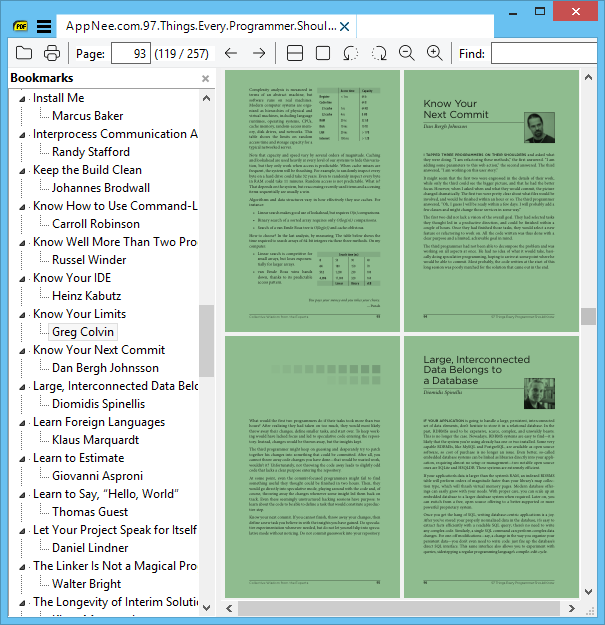
// Table Of Contents //
97 Things Every Programmer Should Know |
- Act with Prudence
- Apply Functional Programming Principles
- Ask “What Would the User Do?” (You Are not the User)
- Automate Your Coding Standard
- Beauty Is in Simplicity
- Before You Refactor
- Beware the Share
- The Boy Scout Rule
- Check Your Code First before Looking to Blame Others
- Choose Your Tools with Care
- Code in the Language of the Domain
- Code Is Design
- Code Layout Matters
- Code Reviews
- Coding with Reason
- A Comment on Comments
- Comment Only What the Code Cannot Say
- Continuous Learning
- Convenience Is not an -ility
- Deploy Early and Often
- Distinguish Business Exceptions from Technical
- Do Lots of Deliberate Practice
- Domain-Specific Languages
- Don’t Be Afraid to Break Things
- Don’t Be Cute with Your Test Data
- Don’t Ignore that Error!
- Don’t Just Learn the Language, Understand its Culture
- Don’t Nail Your Program into the Upright Position
- Don’t Rely on “Magic Happens Here”
- Don’t Repeat Yourself
- Don’t Touch that Code!
- Encapsulate Behavior, not Just State
- Floating-point Numbers Aren’t Real
- Fulfill Your Ambitions with Open Source
- The Golden Rule of API Design
- The Guru Myth
- Hard Work Does not Pay Off
- How to Use a Bug Tracker
- Improve Code by Removing It
- Install Me
- Inter-Process Communication Affects Application Response Time
- Keep the Build Clean
- Know How to Use Command-line Tools
- Know Well More than Two Programming Languages
- Know Your IDE
- Know Your Limits
- Know Your Next Commit
- Large Interconnected Data Belongs to a Database
- Learn Foreign Languages
- Learn to Estimate
- Learn to Say “Hello, World”
- Let Your Project Speak for Itself
- The Linker Is not a Magical Program
- The Longevity of Interim Solutions
- Make Interfaces Easy to Use Correctly and Hard to Use Incorrectly
- Make the Invisible More Visible
- Message Passing Leads to Better Scalability in Parallel Systems
- A Message to the Future
- Missing Opportunities for Polymorphism
- News of the Weird: Testers Are Your Friends
- One Binary
- Only the Code Tells the Truth
- Own (and Refactor) the Build
- Pair Program and Feel the Flow
- Prefer Domain-Specific Types to Primitive Types
- Prevent Errors
- The Professional Programmer
- Put Everything Under Version Control
- Put the Mouse Down and Step Away from the Keyboard
- Read Code
- Read the Humanities
- Reinvent the Wheel Often
- Resist the Temptation of the Singleton Pattern
- The Road to Performance Is Littered with Dirty Code Bombs
- Simplicity Comes from Reduction
- The Single Responsibility Principle
- Start from Yes
- Step Back and Automate, Automate, Automate
- Take Advantage of Code Analysis Tools
- Test for Required Behavior, not Incidental Behavior
- Test Precisely and Concretely
- Test While You Sleep (and over Weekends)
- Testing Is the Engineering Rigor of Software Development
- Thinking in States
- Two Heads Are Often Better than One
- Two Wrongs Can Make a Right (and Are Difficult to Fix)
- Ubuntu Coding for Your Friends
- The Unix Tools Are Your Friends
- Use the Right Algorithm and Data Structure
- Verbose Logging Will Disturb Your Sleep
- WET Dilutes Performance Bottlenecks
- When Programmers and Testers Collaborate
- Write Code as If You Had to Support It for the Rest of Your Life
- Write Small Functions Using Examples
- Write Tests for People
- You Gotta Care about the Code
- Your Customers Do not Mean What They Say
|
97 Things Every Programmer Should Know – Extended |
- Abstract Data Types
- Acknowledge (and Learn from) Failures
- Anomalies Should not Be Ignored
- Avoid Programmer Churn and Bottlenecks
- Balance Duplication, Disruption, and Paralysis
- Be Stupid and Lazy
- Become Effective with Reuse
- Better Efficiency with Mini-Activities, Multi-Processing, and Interrupted Flow
- Code Is Hard to Read
- Consider the Hardware
- Continuous Refactoring
- Continuously Align Software to Be Reusable
- Data Type Tips
- Declarative over Imperative
- Decouple that UI
- Display Courage, Commitment, and Humility
- Dive into Programming
- Don’t Be a One Trick Pony
- Don’t Be too Sophisticated
- Don’t Reinvent the Wheel
- Don’t Use too Much Magic
- Done Means Value
- Execution Speed versus Maintenance Effort
- Expect the Unexpected
- First Write, Second Copy, Third Refactor
- From Requirements to Tables to Code and Tests
- How to Access Patterns
- Implicit Dependencies Are also Dependencies
- Improved Testability Leads to Better Design
- In the End, It’s All Communication
- Integrate Early and Often
- Interfaces Should Reveal Intention
- Isolate to Eliminate
- Keep Your Architect Busy
- Know When to Fail
- Know Your Language
- Learn the Platform
- Learn to Use a Real Editor
- Leave It in a Better State
- Methods Matter
- The Programmer’s New Clothes
- Programmers Are Mini-Project Managers
- Programmers Who Write Tests Get More Time to Program
- Push Your Limits
- QA Team Member as an Equal
- Reap What You Sow
- Respect the Software Release Process
- Restrict Mutability of State
- Reuse Implies Coupling
- Scoping Methods
- Simple Is not Simplistic
- Small!
- Soft Skills Matter
- Speed Kills
- Structure over Function
- Talk about the Trade-offs
- There Is Always Something More to Learn
- There Is No Right or Wrong
- There Is No Such Thing as Self-Documenting Code
- The Three Laws of Test-Driven Development
- Understand Principles behind Practices
- Use Aggregate Objects to Reduce Coupling
- Use the Same Tools in a Team
- Using Design Patterns to Build Reusable Software
- Who Will Test the Tests Themselves?
- Work with a Star and Get Rid of the Truck Factor
- Write a Test that Prints PASSED
- Write Code for Humans not Machines
|
// Download URLs //
(Homepage)
|
If some download link is missing, and you do need it, just please send an email (along with post link and missing link) to remind us to reupload the missing file for you. And, give us some time to respond. |
|
If there is a password for an archive, it should be "appnee.com". |
|
Most of the reserved downloads (including the 32-bit version) can be requested to reupload via email. |